๐ฅ AWE_Sheet - The Excel Powerhouse
AWE_Sheet is a FREE Excel VBA class module, developed with 30+ years of OOP expertise and GPT-4 (AI) collaboration, built to break VBA’s limits and redefine automation. It runs 50% faster than AutoFilter, scales beyond 1M+ rows, and eliminates the need for rewrites—just results, no clutter. Whether your project is a beast or waiting to become one, AWE_Sheet empowers you to tame it!
๐ก Battle-tested in the world’s largest enterprise environments, AWE_Sheet runs faster, cleaner, and more reliably—so sheet structural changes never break your code and force rewrites. AWE_Sheet seamlessly adapts to changes in worksheet structures:
โ Dynamic sheets โ Protected sheets โ Tables โ Shared workbooks โ Network workbooks
๐ For Every Experience Level:
โ Beginner? Learn macros fast and become a power user overnight.
โ Pro? Solve business problems faster—without losing VBA’s native capabilities.
๐ Full source code available. You're in control. Tame your beast. ๐
Jump to: Quick Guide | Start Here | User Guide | Advanced Topics | FAQs | Feedback
Quick Guide – AWE_Sheet at a Glance
This guide covers essential functions and examples to get you automating faster. Learn how to filter, look up data, and more with simple, one-line VBA calls.
Functionality | Example |
---|---|
๐ Initialize & Load Workbooks
Instantly open Simultaneous SharePoint, shared, network, or local workbooks-including already open ones. One command - zero complexity. |
' Initialize a Worksheet in ThisWorkbook. Header is on row 3 Dim aweSh As New AWE_Sheet aweSh.Initialize "SheetName", 3 ' Initialize a network workbook sheet in readonly mode aweSh.Initialize "SheetName", 3, "C:\Path\To\Workbook.xlsx", True ' Initialize a SharePoint workbook aweSh.Initialize "SheetName", 3, "https://sharepoint.com/sites/mysite/Documents/Workbook.xlsx" |
๐ SmartFilter & ๐ SmartFilterRows Filters Protected SheetsโVBA Canโt Locate & filter massive datasets instantlyโ50% FASTER than Autofilter. Works on protected sheets, shared workbooks, and tables. Returns matching row numbers instantlyโNO LOOPS required to find rows. |
Dim aweSh As New AWE_Sheet Dim rowNbr As Variant aweSh.Initialize "Timecard", 3 ' Apply a filter to find matching rows aweSh.SmartFilter "ProjectID", "=Prj-171" aweSh.SmartFilter "Date", ">=1/1/2021", "<=1/31/2021", xlAnd ' Retrieve matching row count (no loops needed to find them) Debug.Print "Matching Row Count: " & aweSh.SmartFilterRows.Count ' Iterate through already found, filtered rows For Each rowNbr In aweSh.SmartFilterRows() Debug.Print "Processing ROW: " & rowNbr Next rowNbr ' Clear the filter for re-use if needed aweSh.SmartFilterClear |
โก SmartCells โ Retrieve Data Dynamically
Goodbye static column numbers. Hello DYNAMIC LOOKUPS. |
Dim aweSh As New AWE_Sheet, rng As Range aweSh.Initialize "Timecard", 3 ' Return the Employee Name located on Row 5 Debug.Print aweSh.SmartCells(4, "Employee Name").Value ' Lookup and return the row where Employee Name = "Name-0001" Set rng = aweSh.SmartCells("Name-0001", "Employee Name") Debug.Print "Name-0001 was found on row: " & rng.row |
๐ SmartCells โ Fast, Multi-Column Lookups
Forget slow, one-cell lookups. FETCH ENTIRE ROWS in a single call. LIGHTNING FAST LOOKUPS on any column, any order, any size. ZERO LOOPS. ZERO CLUTTER. Your code, Your way. |
Dim aweSh As New AWE_Sheet, rowCells As Object aweSh.Initialize "Timecard", 3 ' One Call, One VariableโInstant Multi-Cell Lookups with Dictionary Precision. Set rowCells = aweSh.SmartCells("Name-0001", "Employee Name", _ Array("Date", "Hours", "Revenue")) If rowCells.Count > 0 Then Debug.Print rowCells("Employee Name").row & " | " & _ rowCells("Employee Name").Value & " | " & _ Format(rowCells("Date").Value, "mm/dd/yyyy") & " | " & _ rowCells("Hours") & " | " & _ rowCells("Revenue").Value End If |
๐ Reliable WORKSHEET METADATA
ACCURATE METADATA across all structuresโstandard sheets, tables, filtered data, hidden, and non-congruent rows. BUILD CODE YOU CAN TRUST. |
Dim aweSh As New AWE_Sheet ' Initialize worksheet and extract metadata aweSh.Initialize "Timecard", 3 ' Retrieve worksheet metadata and print results Debug.Print "--- Sheet Metadata ---" & vbLf & _ " Header Row: " & aweSh.HeaderRowNumber & vbLf & _ " Last Row: " & aweSh.LastRowNumber & vbLf & _ " Last Column: " & aweSh.LastColumnNumber & vbLf & _ " Header Range: " & aweSh.HeaderRowRangeX.Address & vbLf & _ " DataBody Range: " & aweSh.DataBodyRangeX.Address & vbLf & _ " Header & Databody: " & aweSh.RangeX.Address & vbLf & _ " Is Table: " & IIf(aweSh.IsTable, "Yes", "No") & vbLf & _ " Is Sheet Empty: " & IIf(aweSh.IsSheetEmpty, "Yes", "No") & vbLf & _ "SmartFilter Active: " & IIf(aweSh.IsSmartFilterActive, "Yes", "No") & vbCrLf & _ " Row Count: " & aweSh.RowCount & vbLf & _ " Column Names: " & Join(aweSh.ColumnNames, ", ") & vbCrLf '------------------------------------------------------------------- ' AWE_Sheet ensures worksheet accuracy in all structures: ' - Works with tables (ListObjects) and standard ranges. ' - Handles non-contiguous rows and dynamic layouts. ' - Eliminates hardcoded referencesโmetadata updates automatically. '------------------------------------------------------------------- |
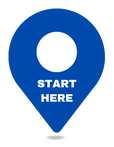
Start Here - See What You've Been Missing
Setting up AWE_Sheet is quick and easy. Download, integrate, and start automating in just a few steps. Follow this guide to unlock its full power and streamline your workflow.
Download & Set Up AWE_Sheet๐ฅ
1๏ธโฃ Download & Extract
- Get the .zip file (class module + example workbook).
- Extract it to access:
- AWE_Sheet_Example.xlsm – Test AWE_Sheet’s features.
- AWE_Sheet.cls – The class module for your projects.
2๏ธโฃ Enable Macros & Explore
- Open AWE_Sheet_Example.xlsm and enable macros.
- Press Alt + F11 to open the VBA editor and step through the code.
๐ Learn how to: Enable Macros | Import VBA class modules
Jump to: Quick Guide | Start Here | User Guide | Advanced Topics | FAQs | Feedback
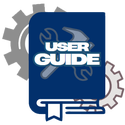
User Guide
AWE_Sheet simplifies Excel VBA so you can meet your deadlines with faster, leaner, and more reliable code. This guide covers everything you need—function breakdowns, examples, and notes. Jump to any section, grab an example, and get back to coding.
Table of Contents by Category:
๐Initialization: Initialize | IsWorkbookOpen | MapColumnNumbers
๐Data Optimization: DeleteEmptyEndRows | EmptyEndRowDetection
๐Metadata: DataBodyRangeX | HeaderRowNumber | HeaderRowRangeX | IsSheetEmpty | IsTable | LastColumnNumber | LastRowNumber | ParentTable | ParentWorkbook | ParentWorksheet | RangeX | RowCount
๐Columns: ColumnNames | ColumnsX | GetColumnNumber | GetUniqueColumnArray | IsInUniqueColumnArray
๐Search: SmartCells | IsSmartFilterActive | SmartFilter | SmartFilterClear | SmartFilterRows | SmartLookup | SmartLookupSort | SmartLookupRows
๐Error Handling: CStateType | RaiseError
Understanding Functions Ending in "X" - Functions ending in X are enhanced versions of native VBA counterparts. They retain familiar functionality but extend their capabilities. See their definitions in the User Guide for details.
Jump to: Quick Guide | Start Here | User Guide | Advanced Topics | FAQs | Feedback
Initialization Functions
Initialize
Description | Prepares the AWE_Sheet object for workbook and worksheet operations. Validates inputs, initializes metadata, and integrates tables (ListObjects ) when present. |
Parameters |
โ
SheetNameOrObj (Variant) โ Target sheet.
โ HeaderRowNumber (Long, Optional) โ Header row if no table is found. Default: 1 .โ WorkbookFileNameOrObj (Variant, Optional) โ Workbook reference.
โ OpenReadOnly (Boolean, Optional) โ If True , opens the workbook in read-only mode. Default: False .
|
Returns | None |
Examples |
๐ Example 1: Initialize in ThisWorkbook mySheet.Initialize "SheetName" ๐ Example 2: Initialize with Workbook File Path (Read-Only) mySheet.Initialize "SheetName", , "C:\Path\To\Workbook.xlsx", True ๐ Example 3: Initialize with SharePoint URL mySheet.Initialize "SheetName", 1, "https://sharepoint.com/sites/mysite/Documents/Workbook.xlsx"
|
Notes |
โ
If a table (ListObject ) exists, the first one is used by AWE_Sheet functions.
|
IsWorkbookOpen
Description | Checks if a workbook is already open in Excel. Helps avoid duplicate openings by verifying if the file is in the active workbooks collection. |
Parameters |
โ
sFullFileName - Full file path of the workbook (e.g., "C:\Files\Workbook.xlsx" ) โ wb - (ByRef and Optional) returns a reference to the workbook if open.
|
Returns | Boolean - True if the workbook is open, False otherwise. |
Examples |
๐ Example: Check if a Workbook is Open isOpen = aweSh.IsWorkbookOpen("C:\Files\Workbook.xlsx", wb)
|
Notes |
โ
Case-insensitive workbook name comparison. โ Only checks workbooks in the current Excel instance. โ Use the wb parameter to reference the open workbook directly.
|
MapColumnNumbers
Description | Maps column names to their respective column numbers from the header row. Validates metadata, ensures unique column names, and populates an internal dictionary for fast lookups. |
Parameters | None |
Error Handling |
โ
State_HeaderColumn (1007) โ Raised if duplicate column names are detected. |
Notes |
โ
Clears existing mappings before creating new ones. โ Validates metadata and ensures unique column names. โ Raises an error for duplicate or empty column names. โ MapColumnNumbers can be called directly to refresh mappings when headers change. โ Initialize automatically invokes MapColumnNumbers but also refreshes all sheet metadata.
|
Examples |
๐ Example: MapColumnNumbers - Update the internal column mapping dictionary mySheet.MapColumnNumbers
|
Data Optimization
DeleteEmptyEndRows
Description |
Detects and removes trailing empty rows ("ghost rows") from the worksheet to ensure UsedRange and DataBodyRangeX reflect actual data boundaries.
|
Returns |
โ
Boolean โ Returns True if ghost rows were successfully deleted or none existed.โ Returns False if ghost rows exist but were skipped by the user.
|
Parameters |
Optional PromptUser As Boolean = True โ If True , the user will be prompted to confirm deletion when ghost rows are detected.If the user selects Cancel, all processing halts via RaiseError .
|
Examples |
๐ Example: Prompt user before deleting empty end rowsDebug.Print mySheet.DeleteEmptyEndRows ๐ Example: Run in silent mode (no user prompt) Debug.Print mySheet.DeleteEmptyEndRows(False)
|
Notes |
โ
When PromptUser is True , the prompt displays the exact range of ghost rows for review.โ If ghost rows are detected and the user clicks Cancel, the function raises a structured error and aborts processing. โ After calling this function, set EmptyEndRowDetection = False to prevent automatic deletion later. |
EmptyEndRowDetection
Description | Controls whether DeleteEmptyEndRows is automatically triggered to remove trailing empty rows.
When enabled, DataBodyRangeX dynamically excludes empty end of sheet rows. |
Get/Set |
โ
Get: Returns the current setting for Empty End Row detection. โ Let: Enables ( True ) or disables (False ) Empty End Row detection.
|
Examples |
๐ Example: Check if Empty End Row Detection is Enabled Debug.Print mySheet.EmptyEndRowDetection
๐ Example: Disable Empty End Row Detection mySheet.EmptyEndRowDetection = False
|
Notes |
โ
Set EmptyEndRowDetection to False after DeleteEmptyEndRows to improve performance. |
Metadata Functions
DataBodyRangeX
Description |
Returns the entire data range of the worksheet below the header row. Supports both tables and standard
ranges, including contiguous and non-contiguous ranges.
While DataBodyRangeX functions like its ListObject counterpart, DataBodyRange, it also works on standard sheets and ensures the last true data row is accurately retrieved. |
Parameters | โ
RaiseSheetEmptyError (Boolean, Optional) โ If True , raises an error if the sheet is empty. Default: False . |
Returns | Range โ The data range of the worksheet, excluding the header row. Returns Nothing if no data rows are available. |
Error Handling |
โ
State_SheetData (1009) โ Raised if no valid data rows are found and RaiseSheetEmptyError is set to True .
|
Notes |
โ
Supports both ListObjects and standard worksheet ranges. โ Handles contiguous, non-contiguous, visible, and non-visible ranges. โ Calculates LastRowNumber dynamically to determine the extent of the data range. โ Removes empty trailing rows (ghost rows) to ensure accurate range detection. โ Does not handle filtered rows โ Instead, use SmartFilter with SmartFilterRows to retrieve AWE_Sheet Filtered rows.
|
Examples |
๐ Example: Print the Data Body Range Address Debug.Print mySheet.DataBodyRangeX.Address
|
HeaderRowNumber
Description | Returns the header row number for the initialized worksheet. |
Parameters | None |
Returns | Long โ The row number of the header row for the initialized worksheet. |
Examples |
๐ Example: Print the Header Row Number Debug.Print mySheet.HeaderRowNumber
|
HeaderRowRangeX
Description | Returns the header row range for the initialized worksheet. |
Parameters | None |
Returns | Range โ The range object representing the header row of the initialized worksheet. |
Examples |
๐ Example: Print the Header Row Range Address Debug.Print mySheet.HeaderRowRangeX.Address
|
IsSheetEmpty
Description | Returns True if the worksheet is empty; otherwise, returns False . |
Parameters | None |
Returns | Boolean โ Returns True if the worksheet is empty, False otherwise. |
Examples |
๐ Example: Check if the Worksheet is Empty Debug.Print mySheet.IsSheetEmpty
|
IsTable
Description | Returns True if the sheet is based on a Table (ListObject); otherwise, returns False . |
Parameters | None |
Returns | Boolean โ Returns True if the sheet is based on a Table (ListObject), False otherwise. |
Examples |
๐ Example: Print if there is a table on the worksheet Debug.Print mySheet.IsTable
|
Notes |
โ
Designed for use with properly initialized sheets as part of the AWE_Sheet workflow. โ Useful for conditional logic when specific table operations are required. |
LastColumnNumber
Description | Retrieves the last header column number in the worksheet. Returns 1 if the worksheet is empty. |
Parameters | None |
Returns | Long โ The header column number. Returns 1 if the worksheet is empty. |
Examples |
๐ Example: Print the Last Populated Column Number Debug.Print mySheet.LastColumnNumber
|
Notes |
โ
Returns 1 if the worksheet is empty, including when the header row is blank. โ Call MapColumnNumbers before calling to dynamically adjust to column changes.
|
LastRowNumber
Description | Retrieves the last valid row number in the worksheet. If no valid data rows exist, it returns the header row number. |
Parameters | None |
Returns | Long โ The last valid row number in the worksheet. If no valid rows are found, it returns the header row number. |
Examples |
๐ Example: Print the Last Valid Row Number Debug.Print mySheet.LastRowNumber
|
Notes |
โ
Determines the last row by calling DataBodyRangeX .โ If no data is present, it defaults to returning the header row number. |
ParentTable
Description | Retrieves the worksheet's associated table (ListObject ) that was identified by the Initialize Function. If a sheet contains multiple tables, the first ListObject found is used. |
Parameters | None |
Returns | ListObject โ The first ListObject (table) on the worksheet. Returns Nothing if no table is found. |
Examples |
๐ Example: Print the Parent Table Name Debug.Print mySheet.ParentTable.Name
|
Notes |
โ
Retrieves the first table (ListObject ) associated with the worksheet. โ Returns Nothing if the worksheet has no table. |
ParentWorkbook
Description | Retrieves the workbook object assigned during the Initialize Function. |
Parameters | None |
Returns | Workbook โ The associated workbook object. Ensures the workbook is valid before returning the reference. |
Error Handling | โ
Raises a State_Workbook error if the workbook is not valid. |
Examples |
๐ Example: Print the Parent Workbook Name Debug.Print mySheet.ParentWorkbook.Name
|
Notes |
โ
Use this property to safely access the workbook associated with the AWE_Sheet instance. |
ParentWorksheet
Description | Retrieves the worksheet object assigned during the Initialize Function. |
Parameters | None |
Returns | Worksheet โ The associated worksheet object. Ensures the worksheet is valid before returning the reference. |
Error Handling | โ
Raises AWE_StateType.State_Worksheet error if the worksheet is not properly initialized or is invalid. |
Examples |
๐ Example: Print the Parent Worksheet Name Debug.Print mySheet.ParentWorksheet.Name
|
Notes |
โ
Use this property to safely access the worksheet associated with the AWE_Sheet instance. |
RangeX
Description |
Retrieves the combined range of the header row and the data body range, adjusting based on the worksheet's structure.
RangeX functions like ListObject.Range, returning a structured dataset that includes the header row and data body range. However, unlike ListObject.Range, RangeX also works with standard worksheet ranges, dynamically determining the dataset structure. |
Parameters | None |
Returns | Range โ The combined range of the header row and data body range. If the worksheet is empty, only the header row range is returned, or A1 if no headers exist. |
Examples |
๐ Example: Print the Combined Header and Data Body Range Debug.Print mySheet.RangeX.Address
|
Notes |
โ
Combines the header row and data body range for convenient access to the entire dataset. โ Updates dynamically when worksheet data changes. |
RowCount
Description | Returns the number of rows in the worksheet's data body range. If no data rows exist, it returns 0 . |
Parameters | None |
Returns | Long โ The total number of rows in the data body range. Returns 0 if no rows are present. |
Examples |
๐ Example: Print the Total Row Count Debug.Print mySheet.RowCount
|
Column Functions
ColumnNames
Description | Retrieves an array of column names from the header row. |
Parameters | None |
Returns | Variant โ An array of column names from the header row. |
Examples |
๐ Example: Print All Column Names Debug.Print Join(mySheet.ColumnNames, ", ")
|
Notes |
โ
Column names are derived from the header row during or the Initialize or MapColumnNumbers functions. โ Useful for iterating over columns dynamically without hardcoding their names. |
ColumnsX
Description |
Retrieves a combined range of specified columns (contiguous or non-contiguous) by name or index.
ColumnsX functions like its VBA counterpart, Columns, but with enhanced flexibility. While Columns references entire columns, ColumnsX retrieves only the data range of specified columns. ColumnsX allows referencing by name or index and supports retrieving multiple columns (contiguous or non-contiguous) as a single range in a single call. |
Parameters |
โ
ColIdxOrName (ParamArray) โ A list of column identifiers:ย ย โข String - The column name (e.g., "Age" )ย ย โข Long - The column index (e.g., 3 )
|
Returns | Range โ A combined range consisting of all specified columns. |
Error Handling |
โ
Raises AWE_StateType.State_SheetData error if the column range cannot be retrieved.
|
Examples |
๐ Example: Print the Address of Selected Columns Debug.Print mySheet.ColumnsX("Age", "Salary").Address
|
Notes |
โ
Supports retrieving multiple columns dynamically, either by name or index. โ Returns a contiguous or non-contiguous range based on the specified columns. |
GetColumnNumber
Description | Resolves the column number from a column name, index, or range. |
Parameters |
โ
col (Variant) โ The column identifier, which can be:
|
Returns | Long โ The resolved column number. |
Error Handling |
โ
Raises AWE_StateType.State_HeaderColumn error in the following cases:
|
Examples |
๐ Example: Retrieve Column Number by Name, Index, or Range
Debug.Print mySheet.GetColumnNumber("Name")
|
Notes |
โ
Handles column identifiers dynamically, allowing flexibility in referencing columns by name, index, or range. โ Raises descriptive errors for unsupported types or missing columns to aid debugging. |
GetUniqueColumnArray
Description | Returns an array of unique, non-blank values from a single column. This function performs a fast in-memory scan and removes duplicates using case-insensitive comparison. |
Parameters |
โ
ColIdxOrName (Variant) โ The column to extract unique values from.
|
Returns | โ Variant โ A 1D array of unique, non-blank values. |
Examples |
๐ Example: Get Unique Project IDs
Dim values As Variant
๐ Example: Loop Through Unique Entries
Dim v
|
Notes |
โ
Blank or empty values are excluded. โ Comparisons are case-insensitive. โ Returns values in the order they are first encountered. |
IsInUniqueColumnArray
Description |
Checks whether a given value exists in an array returned by GetUniqueColumnArray .
Supports exact and wildcard lookups using * and ? for flexible matching.
|
Parameters |
โ
srchStr (Variant) โ The value to search for. Supports wildcards. โ arr (Variant) โ A 1D array of unique values, typically from GetUniqueColumnArray .
|
Returns |
โ
Boolean โ True if the search value exists in the array; otherwise False .
|
Examples |
๐ Example: Check for Exact Match
Dim exists As Boolean
๐ Example: Use Wildcards in Search
exists = mySheet.IsInUniqueColumnArray("PRJ*", mySheet.GetUniqueColumnArray("ProjectID"))
|
Notes |
โ
Supports wildcard characters: * (any characters) and ? (single character). โ Comparison is case-insensitive. โ Leading/trailing spaces in the search string are ignored. |
Search Functions
SmartCells
Description |
Retrieves a range object or dictionary based on row criteria and specified search/return columns.
Supports searching by row number, range reference, or lookup value.
While SmartCells operates like its VBA counterpart, Cells, SmartCells expands functionality by allowing column name lookups, row value searches (lookups), and structured data extraction. SmartCells can return a single-cell range, multiple column values, or an entire row as a dictionary (without the need for additional variables), making SmartCells dynamic in working with spreadsheets and simplifying code. |
Parameters |
โ
rowCriteria (Variant) โ Identifies the target row.
โ srchCol (Variant) โ (Required for lookups) The column to search in.
rowCriteria is a Range , SmartCells will attempt to infer srchCol from the header row position.
โ rtrnCol (Variant, Optional) โ Specifies which columns to return.
โ RaiseSearchError (Boolean, Optional) โ If True , raises an error if no match is found. Default: True .
|
Returns |
โ
Range โ If retrieving a single value. โ Range โ If rtrnCol is empty, returns the cell at the intersection of rowCriteria and srchCol . โ Dictionary โ If retrieving multiple columns or all columns in a row. |
Error Handling |
โ
Raises AWE_StateType.State_Search (1011) if no match is found and RaiseSearchError = True . โ Raises AWE_StateType.State_Parameter (1010) if rowCriteria is unsupported. โ Raises AWE_StateType.State_HeaderColumn (1007) if rtrnCol references a missing column.
|
Examples |
๐ Example: Retrieve a Single Cell Value
Debug.Print mySheet.SmartCells(25, 1).Value ย ย ย ' Retrieve Row 25, Col 1Debug.Print mySheet.SmartCells("Mark Watson",ย "Employee Name").Valueย ย ย ' Find Mark Watson Debug.Print mySheet.SmartCells("Mary Wilson",ย "Employee Name",ย "Email").Valueย ย ย ' Lookup Email for Mary Wilson ๐ Example: Retrieve Multiple Columns as a Dictionary
Dim dict As Object ย ย ย ' Retrieve multiple columns as DictionaryDebug.Print dict("Age").Value & ", " & dict("City").Value ๐ Example: Retrieve All Columns in a Row as a Dictionary
Set dict = mySheet.SmartCells("1001", "ID", "*") ย ย ย ' Retrieve all columns as DictionaryDebug.Print Join(dict.keys, ", ") |
Notes |
โ
Supports searching by row number, range reference, or lookup value. โ If rtrnCol is an array or "*" , returns a dictionary of column-value pairs. โ Raises an error if no match is found and RaiseSearchError = True . โ If rtrnCol is omitted, SmartCells returns the cell at the resolved row and srchCol position. โ If rtrnCol is an empty or invalid array, the function returns Nothing without raising an error.
|
SmartFilter
Description |
Filters rows based on criteria such as text, numbers, or dates. SmartFilter functions like AutoFilter,
its predecessor, but is 50% faster, supports protected sheets, AutoFiltered data, tables,
and standard worksheets, and delivers accurate results on over 1 million rows.
Designed for speed, precision, and scalability, SmartFilter seamlessly handles complex multi-criteria searches
while ensuring high performance. Though SmartFilter functions like AutoFilter, it operates differentlyโit does not filter out or hide rows. Instead, results are stored in memory until retrieved using SmartFilterRows. This design makes SmartFilter faster than AutoFilter and allows it to work on protected sheets. Each SmartFilter call refines the previous results using AND logic between columns. |
Parameters |
โ
srchCol (Variant) โ The column to filter by.
โ criteria1 (Variant) โ The primary filter condition.
โ criteria2 (Variant, Optional) โ A second filter condition for advanced filtering.
criteriaOperator (XlAutoFilterOperator, Optional) โ The logical operator between criteria1 and criteria2 .
โ RaiseSearchError (Boolean, Optional) โ If True , raises an error if no matches are found. Default: True .
|
Examples |
๐ Basic Filtering mySheet.SmartFilter "ProjectID", "=Prj-171" ย ย ย ' Exact match: Prj-171mySheet.SmartFilter "Hours", ">5" ย ย ย ' Greater than 5 hoursmySheet.SmartFilter "Rate", "<=110" ย ย ย ' Less than or equal to 110๐ Wildcard Filtering mySheet.SmartFilter "ProjectID", "Prj*" ย ย ย ' Matches any ProjectID starting with "Prj"mySheet.SmartFilter "ProjectID", "Prj??10" ย ย ย ' Matches "Prj" followed by two characters and "10"๐ Array Filtering mySheet.SmartFilter "Employee Name", Array("Name-0001", "Name-0002", "Name-0003") ย ย ย ' Matches multiple names๐ Multi-Column Filtering โ Each SmartFilter call further narrows the filter results using an AND operator. criteria1 and criteria2 can be combined with xlAnd or xlOr within a single call.
The example below shows how each SmartFilter call is joined using AND. Retrieve the combined filter results using SmartFilterRows() . mySheet.SmartFilter "Hours", ">=5", "<=10", xlAnd ย ย ย ' Hours between 5 and 10mySheet.SmartFilter "ProjectID", "=Prj-158", "=Prj-171", xlOr ย ย ย ' ProjectID is 158 or 171mySheet.SmartFilter "Hours", ">6", "<10", xlAnd ย ย ย ' Hours greater than 6 but less than 10mySheet.SmartFilter "Date", ">3/1/2021", "<3/31/2021", xlAnd ย ย ย ' Filters dates in March 2021 |
Error Handling |
โ
Raises AWE_StateType.State_Parameter (1010) in the following cases:
โ Raises AWE_StateType.State_SheetData (1009) in the following cases:
|
Notes |
โ
Use AutoFilter criteria syntax (> , < , >= , <= , = , <> ) including wildcards (* , ? ). โ criteria1 and criteria2 can be combined using AND (xlAnd ) or OR (xlOr ). โ If criteria1 is an array , then all array criteria are combined using OR (xlOr ). โ If criteria1 is an array , then criteria2 must be empty or a State_Parameter error is raised.โ Each SmartFilter call cumulatively refines the filter using AND. โ Retrieve results using SmartFilterRows() .
|
SmartFilterClear
Description | Clears all filters applied using SmartFilter , resetting stored filter results and allowing new filtering operations to start fresh. |
Parameters | None |
Returns |
โ
Boolean โ Returns True if SmartFilter results were cleared, or False if there were no results to clear.
|
Examples |
๐ Example: Clear SmartFilter Results
mySheet.SmartFilterClear
|
Notes |
โ
Resets all stored row numbers from SmartFilter , ensuring a clean slate for new filters. โ Does not interact with Excelโs AutoFilter or visually remove native filters. โ Use before applying new SmartFilter criteria to prevent unintended filtering constraints. โ Returns True if filters were present and cleared, or False if no filters existed.
|
SmartFilterRows
Description |
Retrieves the row numbers stored by SmartFilter .
If SmartFilter has not yet been called, returns all data rows from the worksheet.
This function does not refilter dataโit strictly returns the previously stored (or default) results for efficient row access.
|
Parameters |
โ
returnRowRanges (Boolean, Optional) โ Specifies whether to return row numbers or row ranges.
|
Returns |
โ
Collection โ A Collection of row numbers or row ranges based on returnRowRanges .
Returns all data rows if no filters have been applied. Returns an empty Collection only if filtering was applied but no rows matched.
|
Examples |
๐ Example: Retrieve Filtered Row Numbers
Dim filteredRows As Collection
Set filteredRows = mySheet.SmartFilterRows
๐ Example: Retrieve Filtered Rows as Row Ranges
Dim rowRanges As Collection
Set rowRanges = mySheet.SmartFilterRows(True)
|
Notes |
โ
Retrieves row numbers or row ranges from stored SmartFilter results. โ Returns a Collection in all cases for easy iteration. โ Returns an empty Collection if no filtered rows exist. โ Works with SmartFilter and does not interact with Excelโs native AutoFilter. โ If SmartFilter has not been called, returns all data rows from the header down.
|
SmartLookup
Description |
Builds a high-performance lookup map using one or more columns as a composite key.
Designed for speed and scale, SmartLookup supports wildcard lookups, optional column formatting,
and sub-second performance even with 100K+ rows.
Use SmartLookupRows to perform lookups against the generated map.
|
Parameters |
โ
lookupMeta (Dictionary, ByRef) โ Output metadata used for future lookups.
Includes:
โ ColIdxOrNames (ParamArray) โ One or more column names or indices.
|
Returns |
โ
Boolean โ True if lookup metadata was successfully created, otherwise False .
|
Examples |
๐ Example: Create a Lookup Using One Column
Dim lookupMeta As Object
๐ Example: Composite Lookup with Formatting
If mySheet.SmartLookup(lookupMeta, "ProjectID", "Date:Format=yyyymmdd") Then
|
Notes |
โ
Returns sub-second performance on 100K+ rows. โ Supports any number of columns as part of the composite key. โ Preserves order and structure for accurate lookups. โ Keys are case-insensitive and use vbTextCompare . โ Use SmartLookupRows to retrieve matches by key.
|
SmartLookupSort
Description |
Sorts the keys used in a SmartLookup dictionary in either ascending or descending order.
Stores the sorted key list in lookupMeta("SortedKeys") for use with SmartLookupRows .
|
Parameters |
โ
lookupMeta (Dictionary, ByRef) โ The metadata object returned by SmartLookup .
Must include the "RootDict" key.
โ sortOrder (XlSortOrder) โ Specifies the sort direction.
|
Returns |
โ
None โ Updates the lookupMeta dictionary in-place by adding the "SortedKeys" entry.
|
Examples |
๐ Example: Sort Lookup Keys in Ascending Order
Call mySheet.SmartLookupSort(lookupMeta, xlAscending)
๐ Example: Sort Lookup Keys in Descending Order
Call mySheet.SmartLookupSort(lookupMeta, xlDescending)
|
Notes |
โ
Stores the result in lookupMeta("SortedKeys") .
โ
Requires a prior call to SmartLookup .
โ
Used in conjunction with SmartLookupRows to return results in sorted order.
โ
Does not modify the original RootDict or underlying data.
|
SmartLookupRows
Description |
Retrieves row numbers matching a composite key from a SmartLookup map.
Supports exact matching and wildcard lookups using * and ? .
Use this function to find matching rows based on one or more key values generated by SmartLookup .
|
Parameters |
โ
lookupMeta (Dictionary, ByRef) โ The lookup metadata generated by SmartLookup .
Must contain the "RootDict" key.
โ ColKeys (Variant) โ An array of key values corresponding to the columns used in the original lookup.
โ FirstOnly (Boolean, Optional) โ If True , returns only the first matching row. Default: False .
|
Returns |
โ
Collection โ If FirstOnly = False , returns a Collection of matching row numbers. โ Long โ If FirstOnly = True , returns the first matching row number or Empty if none found.
|
Examples |
๐ Example: Exact Match
Dim results As Collection
๐ Example: Wildcard Match
Set results = mySheet.SmartLookupRows(lookupMeta, Array("PRJ*", "2025*"))
๐ Example: Return First Match Only
Dim firstRow As Long
|
Notes |
โ
Composite keys must match the order used in the original SmartLookup . โ Wildcards ( * , ? ) apply to the full composite key. โ Matching is case-insensitive when vbTextCompare is used in SmartLookup . โ Use SmartCells with returned row numbers to retrieve cell values.
|
Error Handling Functions
CStateType
Description | Retrieves the numeric value of an AWE_StateType enum, making it useful for debugging and validation.
This function does not raise errorsโit simply returns the corresponding numeric value. |
Parameters |
โ
EnumName (AWE_StateType) โ The state enum to retrieve its numeric value.
|
Returns | โ
Long โ The numeric value corresponding to the provided AWE_StateType enum. Below are enumerated values and definitions:
๐น 1000 : State_Workbook - Workbook is invalid.๐น 1001 : State_ReadOnly - Workbook is read-only.๐น 1002 : State_Worksheet - Worksheet is invalid.๐น 1003 : State_HasData - Worksheet is missing data.๐น 1005 : State_ListObject - ListObject (Table) does not exist or is invalid.๐น 1006 : State_HeaderRow - Header row is invalid.๐น 1007 : State_HeaderColumn - Header column could not be found.๐น 1008 : State_Protection - Workbook or worksheet is protected.๐น 1009 : State_SheetData - Data on the sheet is invalid.๐น 1010 : State_Parameter - Parameter is invalid.๐น 1011 : State_Search - Search is invalid. |
Examples |
๐ Example: Return the enumerated (numeric) equivalent for State_Workbook, i.e. 1000 Debug.Print mySheet.CStateType(State_Workbook)
|
Notes |
โ
Each AWE_StateType enum has a corresponding numeric value. โ Used primarily for debugging and error handling. โ If an error occurs and the queried state is unknown, it is considered invalid. |
RaiseError
Description |
Triggers a structured VBA error with a custom error code, function name, and description.
RaiseError is used for custom validation, process control, and structured error handling in VBA workflows.
Unlike native VBA error handling, this function includes a callstack and accumulated messages, making debugging easier by providing detailed execution context. |
Parameters |
โ
errorCode (Long) โ Numeric error code identifying the issue. โ functionName (String) โ The name of the function where the error occurred. โ description (String, Optional) โ Optional error message providing additional context.
|
Returns | None โ This function does not return a value. It raises an error and halts execution unless handled. |
Examples |
๐ Example: Trigger a Custom Error mySheet.RaiseError 2001, "ValidateInput", "A required field was left empty."
๐ Example: Use RaiseError in a Validation Check
If userInput = "" Then mySheet.RaiseError 3002, "ProcessData", "User input cannot be blank."
|
Common Use Cases |
โ
Enforcing required fields and validating user inputs. โ Preventing invalid data types or unexpected values. โ Implementing custom error handling for business logic. โ Providing clear debugging messages when an issue occurs. |
Notes |
โ
RaiseError is not limited to AWE_Sheetโit is a general-purpose function for structured error handling. โ Errors raised must be handled using standard VBA error handling (e.g., On Error Resume Next ). |
Advanced Topics
The Advanced Topics section is designed for experienced users who want to explore the full potential of AWE_Sheet. Building on the foundational knowledge in the User Guide, these examples focus on advanced workflows for efficient data handling, automation, and integration with Excel-native features.
This section covers:
- Handling large datasets with arrays for in-memory operations.
- Dynamic column mapping and bulk processing for scalable macros.
- Using AWE_Sheet with Excel-native features like conditional formatting and clipboard operations.
- Implementing robust error-handling strategies for complex workflows.
How to Use This Section:
- Start with the User Guide for a strong understanding of AWE_Sheet’s core functionality.
- Explore the advanced examples for optimized solutions to real-world scenarios.
- Combine and adapt these examples to enhance your automation projects.
Jump to: Quick Guide | Start Here | User Guide | Advanced Topics | FAQs | Feedback
Functionality | Example |
---|---|
Error Handling - Ensure stability and structured error management in VBA workflows. | |
Local Error Handling Handles errors within the same function to prevent execution failures. Note: Local error handling allows controlled execution without terminating macros but may lead to silent failures if not properly managed. |
Sub LocalErrorHandling_Example() Dim mySheet As New AWE_Sheet ' Enable local error handling On Error Resume Next ' Attempt to initialize with a missing sheet mySheet.Initialize "NonExistentSheet" ' Apply a SmartFilter on an uninitialized sheet mySheet.SmartFilter "Status", "Completed" ' Check for an error and handle it manually If Err.Number <> 0 Then Debug.Print "Local Error: " & mySheet.CStateType(Err.Number) Err.Clear End If On Error GoTo 0 ' Disable local error handling End Sub |
Propagated Error Handling Errors are raised and handled centrally, preserving the call stack for debugging. Note: This method is preferred for debugging and structured workflows where silent failures are unacceptable. |
Sub PropagatedErrorHandling_Example() On Error GoTo ErrorHandler Dim mySheet As New AWE_Sheet ' Initialize the sheet mySheet.Initialize "SheetWithData" ' Apply a SmartFilter that will trigger an error (State_Search, no matches found) mySheet.SmartFilter "Status", "Does Not Exist" Exit Sub ErrorHandler: Debug.Print "Error encountered: " & Err.Description ' Handle only the most relevant errors Select Case mySheet.CStateType(Err.Number) Case State_Workbook Debug.Print "Error: Invalid workbook." Case State_Worksheet Debug.Print "Error: Invalid worksheet." Case State_Search Debug.Print "Error: No search results found." End Select Exit Sub End Sub |
Bulk Data Operations - Efficiently process large datasets. | |
Bulk Processing - Update Data with Arrays Efficiently process and update large datasets in memory before writing back in a single operation. Note: This example demonstrates a bulk update on a 10K+ row dataset, adjusting Rate and recalculating Revenue for a specific ProjectID .โ Important: Avoid bulk updates on sheets with AutoFilter applied, as data will be incorrectly written back to the sheet. |
Sub BulkUpdate_Project171() Dim mySheet As New AWE_Sheet Dim arrData As Variant Dim projectCol As Long, rateCol As Long, revenueCol As Long, hoursCol As Long Dim i As Long, startTime As Double Const RATE_INCREASE As Double = 10 ' Flat increase in Rate ' Initialize the sheet mySheet.Initialize "Timecard", 3 ' Start timer startTime = Timer ' Load entire sheet data into an array arrData = mySheet.DataBodyRangeX.Value ' Determine column indexes projectCol = mySheet.GetColumnNumber("ProjectID") rateCol = mySheet.GetColumnNumber("Rate") revenueCol = mySheet.GetColumnNumber("Revenue") hoursCol = mySheet.GetColumnNumber("Hours") ' Loop through rows and update Rate & Revenue where ProjectID = "Prj-171" For i = LBound(arrData, 1) To UBound(arrData, 1) If arrData(i, projectCol) = "Prj-171" Then arrData(i, rateCol) = arrData(i, rateCol) + RATE_INCREASE ' Increase Rate arrData(i, revenueCol) = arrData(i, rateCol) * arrData(i, hoursCol) ' Recalc Revenue End If Next i ' Write updated array back to the sheet in one operation mySheet.DataBodyRangeX.Value = arrData ' Output execution time Debug.Print "Bulk update completed in: " & Format(Timer - startTime, "0.000") & " seconds" End Sub |
Advanced Features - Extend functionality with advanced operations and integration. | |
Clipboard-Friendly Data Export Copy SmartFilter results and paste seamlessly. This method leverages Union for efficient row selection.
While reliable for most use cases, performance may degrade beyond
100K+ disjointed rows. Consider batch processing
for extreme cases.
|
Sub CopyFilteredRows_Simple() On Error GoTo ErrorHandler Dim aweSh As New AWE_Sheet, rngToCopy As Range, rng As Variant Dim destSheet As Worksheet ' Initialize and filter aweSh.Initialize "Timecard", 3 aweSh.SmartFilter "ProjectID", "Prj-171" ' Build range with header + filtered rows Set rngToCopy = aweSh.HeaderRowRangeX For Each rng In aweSh.SmartFilterRows(True) Set rngToCopy = Union(rngToCopy, rng) Next rng ' Ensure destination sheet exists On Error Resume Next: Set destSheet = ThisWorkbook.Sheets("Sheet2") On Error GoTo ErrorHandler If destSheet Is Nothing Then Set destSheet = ThisWorkbook.Sheets.Add destSheet.Name = "Sheet2" End If ' Copy and paste all rows ignoring autofilter rngToCopy.Copy destSheet.Range("A1").PasteSpecial xlPasteAll ' === This method ensures all data is copied, regardless of AutoFilter ================= ' Dim area As Range, destCell As Range ' Set destCell = destSheet.Cells(destSheet.Rows.Count, 1).End(xlUp).Offset(1, 0) ' For Each area In rngToCopy.Areas ' area.Copy ' destCell.PasteSpecial xlPasteAll ' Set destCell = destSheet.Cells(destSheet.Rows.Count, 1).End(xlUp).Offset(1, 0) ' Next area ' ===================================================================================== Application.CutCopyMode = False Exit Sub ErrorHandler: Debug.Print "Error: " & Err.Description & " | " & Err.Source End Sub |
FAQs
Frequently Asked Questions
1. What is AWE_Sheet?
AWE_Sheet is an advanced VBA-based tool designed to streamline Excel automation. It extends native VBA functionality with powerful, intuitive commands that simplify workflows, reduce code, and handle dynamic operations effortlessly.
2. Who is AWE_Sheet for?
AWE_Sheet is perfect for:
- Project Managers: Streamline reporting and task tracking.
- Business Analysts: Automate data analysis and insights.
- Developers: Write less code while achieving more.
- Anyone using Excel: Simplify everyday tasks and save time.
3. What makes AWE_Sheet different from VBA?
AWE_Sheet enhances native VBA with:
- Dynamic Flexibility: Functions like CellsX and FilterX handle complex tasks with ease.
- Error Handling: Built-in validations ensure reliable code execution.
- Reduced Complexity and Code: Simplifies repetitive tasks and lets you focus on your solution.
4. Can AWE_Sheet handle large datasets?
Yes! AWE_Sheet is optimized for performance and can efficiently handle thousands of rows, ensuring reliability even with complex operations.
5. What are the “X” functions in AWE_Sheet?
Functions like CellsX, RangeX, and FilterX are extended versions of native VBA functions. They support similar signatures but add powerful features like dynamic handling and robust error validation.
6. Is AWE_Sheet beginner-friendly?
Absolutely! While advanced users will appreciate its power, AWE_Sheet’s intuitive syntax and documentation make it accessible to VBA beginners.
7. Does AWE_Sheet work with protected worksheets?
AWE_Sheet includes robust error messages to notify you if a protected worksheet blocks an operation. Native Excel filtering does not work on protected worksheets, and since AWE_Sheet’s filtering functionality is built on top of Excel’s native filtering, it is also blocked. However, you can use FindRowNumbers as a powerful and fast alternative that works seamlessly, even on protected worksheets.
8. Can AWE_Sheet integrate with SharePoint files?
Yes! AWE_Sheet seamlessly integrates with SharePoint files, opening them in application mode. Use the Initialize method’s OpenReadOnly parameter to specify whether to open files in read-only or writable mode. Note: Remove ":x:/r/" from the SharePoint file path to open the workbook in writable mode.
9. What if I encounter errors while using AWE_Sheet?
AWE_Sheet includes built-in error codes and messages to guide troubleshooting. The User Guide also provides detailed explanations and examples for resolving issues.
10. How do I get started with AWE_Sheet?
Download the .cls file (see How to Import a VBA Class Module) and, if needed, download the example workbook. Then, start with the Quick Reference Guide for an overview of core functions and explore the User Guide for detailed explanations, examples, and best practices.
11. How do I achieve fast performance with AWE_Sheet?
For sub-second performance in filtering and searching, use FilterX and FindRowNumbers. FindRowNumbers works with or without filters and can further refine searches within filtered rows without needing to reset filters. Use CellsX for one-off searches or to retrieve row values after FilterX and FindRowNumbers searches. To future-proof your workflow and minimize costly overhead, avoid looping through rows without FilterX or FindRowNumbers. This workflow ensures efficient operations, even on large worksheets.
12. Can I request new features?
Yes! We value your feedback and encourage you to share feature suggestions or improvement ideas. Use the contact form on our website or leave a comment below to let us know how we can make AWE_Sheet even better.
Feedback and Testimonials
Project Manager
"As a project manager juggling multiple workbooks daily, AWE_Sheet has been a game-changer. The ability to filter, retrieve, and update data with a few intuitive commands has saved me countless hours. I no longer have to worry about complex syntax—AWE_Sheet handles it all effortlessly!"
– Sarah T., Project Manager
Business Analyst
"AWE_Sheet transformed the way I analyze data in Excel. Dynamic column handling and robust error-checking have made my workflows faster and more reliable. I love how easy it is to integrate with both standard sheets and tables—it’s the ultimate tool for any analyst!"
– Michael L., Business Analyst
Macro Developer
"As someone who’s worked with VBA for years, AWE_Sheet feels like a breath of fresh air. Its extended functionality eliminates the need for repetitive code and makes even the most complex operations simple and intuitive. It’s a must-have for any developer working with Excel!"
– David R., VBA Developer
Finance Professional
"Managing large datasets and maintaining dashboards has always been a challenge, but AWE_Sheet makes it seamless. Its ability to easily update tables ensures my dashboards stay accurate and up-to-date. The error-handling capabilities alone give me peace of mind, knowing my reports are reliable every time. Highly recommend this tool for finance teams!"
– Jennifer M., Financial Analyst
Educator
"Teaching Excel automation has become so much easier with AWE_Sheet. Students grasp the concepts quickly because the functions are intuitive and eliminate unnecessary complexity. It’s a powerful tool that bridges the gap between beginners and professionals."
– Dr. Emily C., Data Science Instructor
Add comment
Comments
AWE_Sheet has been a lifesaver for managing my project reports. Can you add functionality to export filtered data directly into a new workbook?